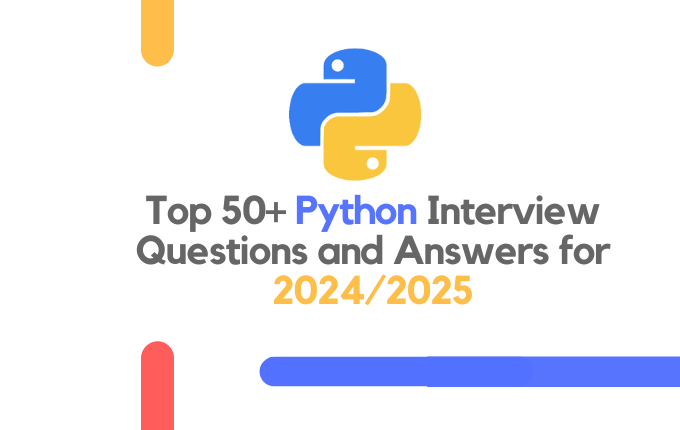
Top 50+ Python Interview Questions and Answers for 2024/2025
Python continues to be one of the most popular programming languages for software development, data science, and machine learning. As companies embrace the power and simplicity of Python, the demand for skilled Python developers continues to rise. If you’re preparing for a Python interview, it’s essential to get ready with the most common and advanced Python interview questions. In this article, we’ve compiled a list of top 50+ Python interview questions and answers for 2024/2025 to help you ace your next interview.
Basic Python Interview Questions
-
What is Python? Answer:
Python is a high-level, interpreted, and general-purpose programming language known for its simplicity and readability. It was created by Guido van Rossum and was first released in 1991. Python is widely used for web development, automation, data analysis, artificial intelligence, scientific computing, and more.Example:
Further reading: Official Python Documentationprint("Hello, World!")
-
What are the key features of Python? Answer:
Key features of Python include:- Easy syntax: Python is known for its clean, readable syntax.
- Dynamic typing: You don’t have to declare the type of a variable when you create it.
- Object-oriented: Supports classes and inheritance.
- Extensive standard library: Python has a vast collection of built-in modules and packages.
- Cross-platform compatibility: Python can run on various platforms like Windows, Linux, and macOS.
-
What are Python’s data types? Answer:
Python supports several built-in data types, including:int
: Integer numbers (e.g.,5
)float
: Decimal numbers (e.g.,3.14
)str
: Strings, used for text (e.g.,"hello"
)list
: Ordered, mutable collection (e.g.,[1, 2, 3]
)tuple
: Ordered, immutable collection (e.g.,(1, 2, 3)
)dict
: Unordered collection of key-value pairs (e.g.,{"key": "value"}
)set
: Unordered collection of unique items (e.g.,{1, 2, 3}
)
Example:
x = 10 # int y = 3.14 # float name = "John" # str my_list = [1, 2, 3] # list my_dict = {"name": "John", "age": 30} # dict
-
What is a list in Python? Answer:
A list is a collection of items in a specific order. Lists are mutable, meaning they can be modified after creation. Lists are defined using square brackets[]
.Example:
my_list = [1, 2, 3] my_list.append(4) # Adding an element to the list print(my_list) # Output: [1, 2, 3, 4]
-
How do you define a function in Python? Answer:
A function in Python is defined using thedef
keyword. Functions can take parameters and return values. The syntax is straightforward and easy to read.Example:
def greet(name): return f"Hello, {name}!" print(greet("Alice")) # Output: Hello, Alice!
Intermediate Python Interview Questions
-
What is a decorator in Python? Answer:
A decorator is a function that modifies the behavior of another function or class. It allows you to extend or alter the functionality of a callable object (like a function) without permanently modifying it.Example:
Further reading: Python decoratorsdef decorator(func): def wrapper(): print("Before function call") func() print("After function call") return wrapper @decorator def say_hello(): print("Hello!") say_hello()
-
What is a lambda in Python? Answer:
A lambda is a small anonymous function defined using thelambda
keyword. It can have any number of arguments but only one expression. Lambda functions are often used for short-term tasks.Example:
multiply = lambda x, y: x * y print(multiply(2, 3)) # Output: 6
-
What is the difference between
deepcopy
andshallow copy
? Answer:- A shallow copy creates a new object, but it does not recursively copy objects within it. The references to objects in the original are preserved.
- A deep copy creates a new object and recursively copies all objects within it, so changes in the copied object do not affect the original.
Example:
import copy original = [[1, 2, 3], [4, 5, 6]] shallow = copy.copy(original) deep = copy.deepcopy(original)
-
What is the
self
keyword in Python? Answer:
Theself
keyword refers to the instance of the class. It is used to access instance variables and methods within a class. It allows you to refer to the object being created or accessed.Example:
class MyClass: def __init__(self, name): self.name = name def greet(self): print(f"Hello, {self.name}!") obj = MyClass("Alice") obj.greet() # Output: Hello, Alice!
-
What are list comprehensions in Python? Answer:
List comprehensions provide a concise way to create lists in Python. They are a more syntactically elegant and compact way to create new lists by applying an expression to each item in an existing iterable.Example:
squares = [x**2 for x in range(5)] print(squares) # Output: [0, 1, 4, 9, 16]
Further reading: List Comprehensions in Python
Advanced Python Interview Questions
-
What is the Global Interpreter Lock (GIL) in Python? Answer:
The Global Interpreter Lock (GIL) is a mutex that allows only one thread to execute Python bytecode at a time. This means that Python threads do not fully utilize multiple processors in multi-core systems for CPU-bound tasks, though they do work well for I/O-bound tasks.Further reading: Understanding the GIL
-
What is the difference between
@staticmethod
and@classmethod
? Answer:@staticmethod
: Defines a method that does not access or modify class or instance data. It works like a normal function but belongs to the class.@classmethod
: Defines a method that takes the class itself as the first argument (cls
) and can modify the class state.
Example:
class MyClass: @staticmethod def static_method(): print("This is a static method.") @classmethod def class_method(cls): print(f"This is a class method of {cls}.") MyClass.static_method() # Output: This is a static method. MyClass.class_method() # Output: This is a class method of <class '__main__.MyClass'>.
-
What is a metaclass in Python? Answer:
A metaclass is a class of a class. It defines how a class behaves and is used to create or modify classes at the time of their creation.Example:
class Meta(type): def __new__(cls, name, bases, dct): dct['class_name'] = name return super().__new__(cls, name, bases, dct) class MyClass(metaclass=Meta): pass obj = MyClass() print(obj.class_name) # Output: MyClass
Python for Data Science & Machine Learning
-
What is NumPy? Answer:
NumPy is a library for numerical computing in Python. It provides support for multi-dimensional arrays and matrices, along with a collection of mathematical functions to operate on these arrays.Example:
Further reading: NumPy Documentationimport numpy as np arr = np.array([1, 2, 3]) print(arr) # Output: [1 2 3]
-
What is Pandas? Answer:
Pandas is a powerful data manipulation and analysis library. It provides two main data structures:DataFrame
(2-dimensional) andSeries
(1-dimensional), which allow handling of structured data.Example:
import pandas as pd data = {'Name': ['Alice', 'Bob'], 'Age': [25, 30]} df = pd.DataFrame(data) print(df)
Further reading: Pandas Documentation
- What is the purpose of
__init__()
method in Python? Answer:
The__init__()
method is a special method in Python used for object initialization. It’s automatically called when a new object of a class is created. This method allows you to set initial values for the object's attributes.
Example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
person = Person("Alice", 25)
print(person.name) # Output: Alice
- What is the difference between
del
andremove
in Python? Answer:
del
: Removes a variable or item from a list by its index or completely deletes a variable.remove
: Removes the first occurrence of a value from a list.
Example:
my_list = [1, 2, 3, 4, 5]
del my_list[2] # Removes element at index 2
print(my_list) # Output: [1, 2, 4, 5]
my_list.remove(4) # Removes element with value 4
print(my_list) # Output: [1, 2, 5]
18. What is the use of the `with
` statement in Python? Answer:
The with
statement is used for resource management, such as file handling. It ensures that resources are automatically cleaned up after their usage, even if an exception occurs.
with open("file.txt", "r") as file:
content = file.read()
print(content)
Further reading: Context Manager in Python
- What is the difference between
is
and==
in Python? Answer:
==
checks for value equality (whether two objects have the same value).is
checks for identity equality (whether two objects are the same instance).
Example:
a = [1, 2, 3]
b = [1, 2, 3]
c = a
print(a == b) # True
print(a is b) # False
print(a is c) # True
- What are Python’s built-in data structures? Answer:
Python provides several built-in data structures:
- List: Ordered, mutable, and allows duplicates.
- Tuple: Ordered, immutable, and allows duplicates.
- Set: Unordered, mutable, and does not allow duplicates.
- Dictionary: Unordered, mutable, and stores key-value pairs.
-
What is the difference between
range()
andxrange()
in Python? Answer:
In Python 2,range()
returns a list, whilexrange()
returns an iterator, which is more memory efficient. In Python 3,xrange()
was removed, andrange()
behaves likexrange()
. -
What is the purpose of the
__str__()
method in Python? Answer:
The__str__()
method is a special method used to define the string representation of an object. It is called whenstr()
orprint()
is applied to an object.
Example:
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def __str__(self):
return f"Person(Name: {self.name}, Age: {self.age})"
person = Person("John", 30)
print(person) # Output: Person(Name: John, Age: 30)
- How does Python handle memory management? Answer:
Python uses automatic memory management, including a garbage collector for reclaiming unused memory. The reference counting system keeps track of objects, and when an object's reference count drops to zero, its memory is freed. Additionally, Python employs a cyclic garbage collector to handle circular references.
Further reading: Memory Management in Python
- What is the difference between
append()
andextend()
in Python? Answer:
append()
: Adds a single element to the end of a list.extend()
: Adds multiple elements (from another iterable) to the end of a list.
Example:
my_list = [1, 2, 3]
my_list.append(4) # Output: [1, 2, 3, 4]
my_list.extend([5, 6]) # Output: [1, 2, 3, 4, 5, 6]
- What is the purpose of
super()
in Python? Answer:super()
is used to call a method from a parent class. It's commonly used in method overriding to extend the functionality of a method in the child class while maintaining the behavior of the parent class.
Example:
class Animal:
def speak(self):
print("Animal speaking")
class Dog(Animal):
def speak(self):
super().speak()
print("Dog barking")
dog = Dog()
dog.speak()
- What are Python generators? Answer:
Python generators are functions that return an iterable set of items, one at a time, using theyield
keyword. Generators allow for lazy evaluation, meaning values are generated only when required, improving performance in cases where not all values need to be processed.
Example:
def my_gen():
yield 1
yield 2
yield 3
for value in my_gen():
print(value)
- What is a module in Python? Answer:
A module is a file containing Python definitions and statements. Modules help organize code into logical components and promote reusability.
Example:
# math_module.py
def add(a, b):
return a + b
You can import the module and use its functions:
44. What are Python's exception types?
Answer:
Python has several built-in exception types, including:
ValueError
: Raised when a function receives an argument of the right type but inappropriate value.IndexError
: Raised when a sequence index is out of range.KeyError
: Raised when a dictionary key is not found.
Example:
try:
x = int("abc")
except ValueError as e:
print("Error:", e) # Output: invalid literal for int() with base 10: 'abc'
45. What is a Python Iterator
?
Answer:
An iterator is an object that can be iterated upon, meaning it returns one item at a time from a collection. It implements two methods: __iter__()
and __next__()
.
Example:
class MyIterator:
def __init__(self, start, end):
self.current = start
self.end = end
def __iter__(self):
return self
def __next__(self):
if self.current < self.end:
self.current += 1
return self.current - 1
else:
raise StopIteration
my_iter = MyIterator(0, 3)
for num in my_iter:
print(num) # Output: 0, 1, 2
46. What is the purpose of __del__()
method in Python?
Answer:
The __del__()
method is a destructor in Python. It is automatically called when an object is about to be destroyed. It’s used to clean up resources, such as closing files or releasing network connections.
Example:
class MyClass:
def __del__(self):
print("Object destroyed")
obj = MyClass()
del obj # Output: Object destroyed
47. What is isinstance()
used for in Python?
Answer:isinstance()
checks whether an object is an instance of a specified class or a tuple of classes.
Example:
x = 5
print(isinstance(x, int)) # Output: True
48. How does Python handle type conversion?
Answer:
Python provides functions for type conversion, such as:
int()
: Converts a value to an integer.float()
: Converts a value to a float.str()
: Converts a value to a string.
Example:
x = "10"
y = int(x)
print(y + 5) # Output: 15
49. How can you create a virtual environment in Python?
Answer:
A virtual environment isolates Python dependencies, ensuring that different projects don't share the same libraries. You can create a virtual environment using the venv
module.
Example:
python3 -m venv myenv
Activate it:
- On Windows:
myenv\Scripts\activate
- On macOS/Linux:
source myenv/bin/activate
50. What is the purpose of globals()
and locals()
in Python?
Answer:
globals()
: Returns a dictionary representing the current global symbol table.locals()
: Returns a dictionary representing the current local symbol table.
Example:
def my_func():
x = 10
print("locals:", locals())
print("globals:", globals())
my_func()
51. How do you handle JSON in Python?
Answer:
You can use the json
module to work with JSON data in Python. It provides functions like json.dumps()
for encoding and json.loads()
for decoding.
Example:
import json
data = {'name': 'Alice', 'age': 25}
json_str = json.dumps(data)
print(json_str) # Output: {"name": "Alice", "age": 25}
parsed_data = json.loads(json_str)
print(parsed_data['name']) # Output: Alice
Bonus Tips for Python Interviews
- Practice coding on platforms like HackerRank to improve problem-solving skills.
- Review Python’s official documentation to familiarize yourself with the latest features and libraries.
- Use version control (Git) to manage your projects and showcase your work to potential employers.
- Stay updated with Python’s latest releases to be aware of improvements, new features, and changes.
Conclusion
Python remains an essential tool for developers in 2024/2025. With the language’s vast applications in web development, data science, and machine learning, mastering Python is crucial for any programmer looking to advance their career. By preparing for these top 50+ Python interview questions, you can be ready for your next job interview, no matter the level of difficulty. Don't forget to check out Python’s official documentation to get deeper insights and additional tips!
0 Comments
Like 1